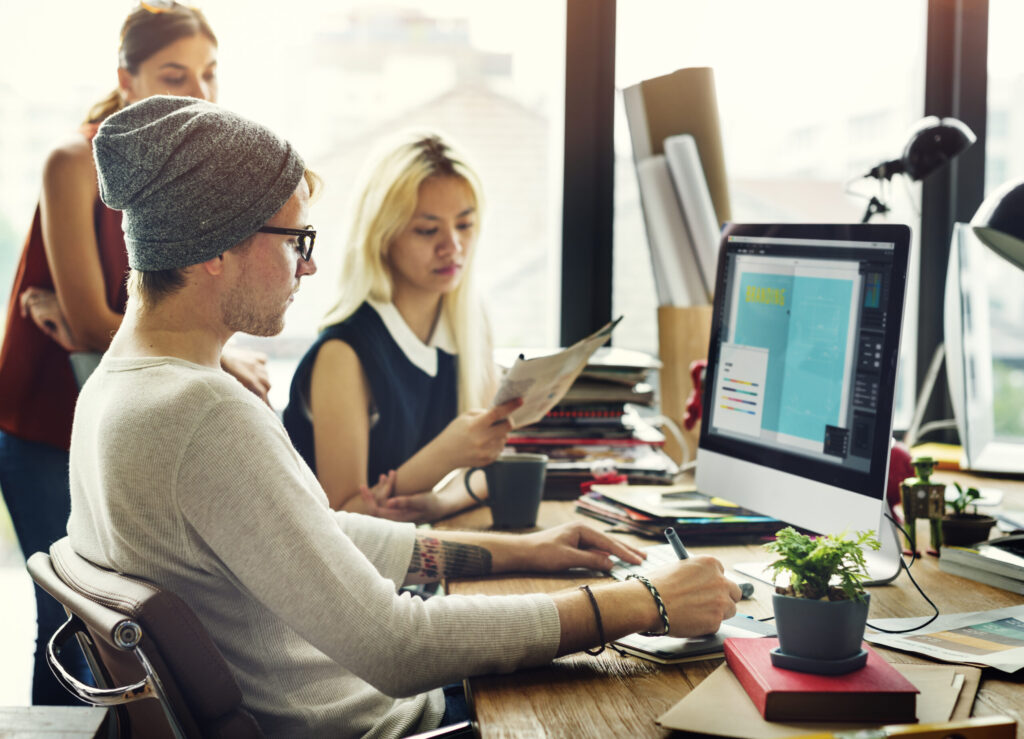
Business Office Connection Contemporary Working Concept
Best Practices for Using Laravel Pipelines
Best Practices for Using Laravel Pipelines
Laravel pipelines offer a robust method for streamlining complex tasks within applications, allowing developers to construct a series of class-based actions that process data in a clear and maintainable sequence. Below, we navigate through the core aspects of Laravel pipelines and how to utilize them effectively in your projects.
Understanding Laravel Pipelines for Efficient Code Management
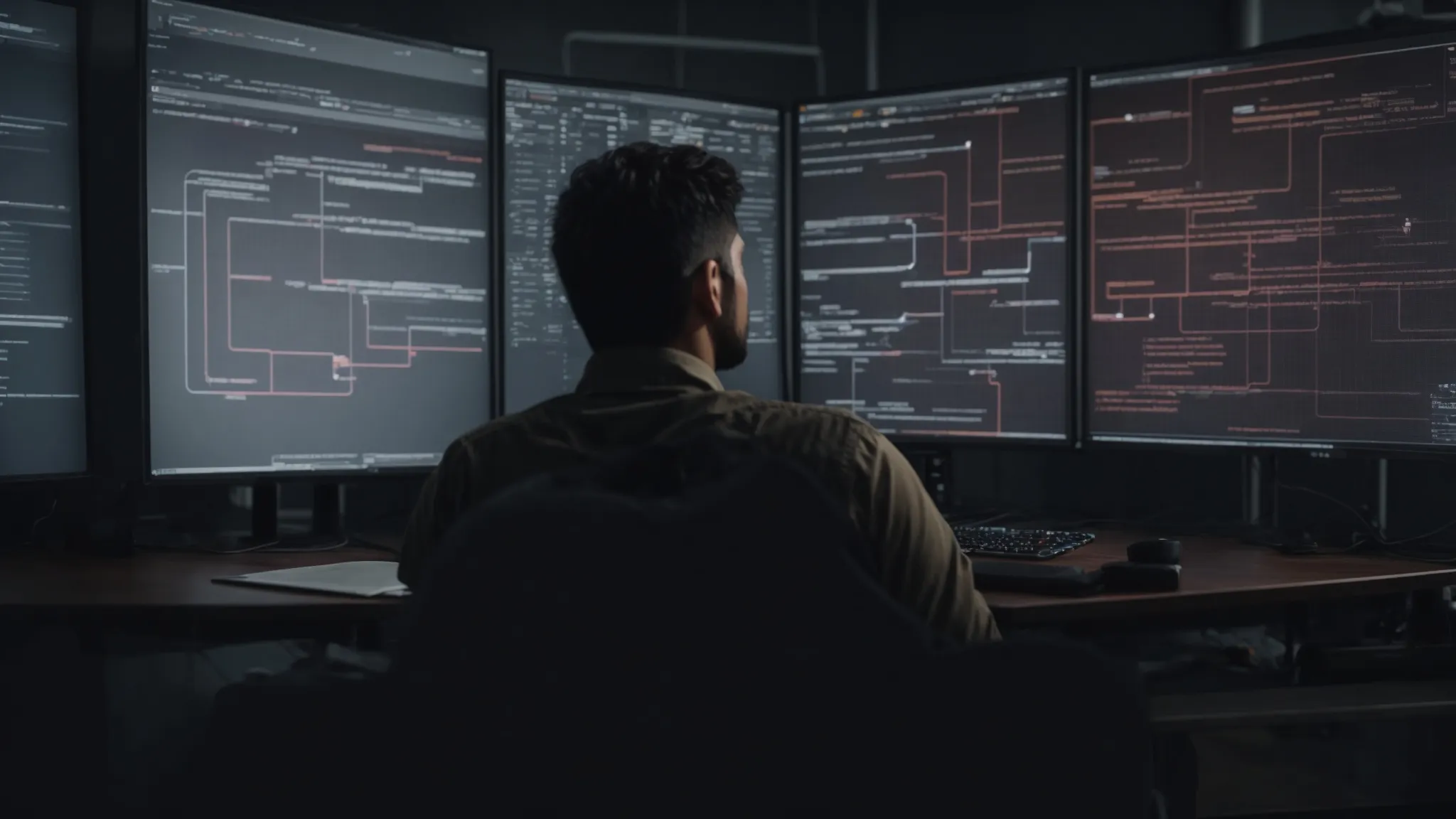
Laravel pipelines are essentially a chain of responsibility pattern implementation, providing a fluent interface to sequence tasks and pass them through a series of classes. They enable developers to break down complex procedures into manageable, logical stages, simplifying the process of debugging and enhancing code readability. This compartmentalization is especially beneficial when dealing with operations that need to be processed sequentially, such as API request handling or data transformation.
Built on the underlying IlluminatePipelinePipeline class, Laravel pipelines work by creating a pipeline instance, which then travels through a series of steps or “pipes.” Each “pipe” represents a callable function or a class that takes an input, processes it, and passes it on to the next ‘pipe’ in the sequence. It’s important to note that although each ‘pipe’ handles part of the task, they work together to complete a common objective.
Understanding the conceptual structure of Laravel pipelines is crucial before diving into implementation. Essentially, they permit the passage of an object through a series of classes and/or callbacks, culminating with the final object’s delivery. The magic happens by passing the object along with a closure that represents the next ‘pipe’. When implemented properly, pipelines can be a powerful tool for keeping code clean and focused. Familiarizing oneself with the mechanics of a Laravel pipeline is the first step toward mastering its application in development.
Leveraging Laravel Pipelines for Data Processing Tasks
Laravel pipelines are particularly advantageous when dealing with data processing tasks. For instance, imagine a scenario where an application must transform incoming data from an API request into a specific format before processing it. Ideally, each transformation step should be handled separately to maintain code clarity and allow for scalability.
In such cases, pipelines serve as an assembly line for the data, where each transformation represents a different segment of the assembly line. By doing this, developers can isolate parts of the processing task, thereby making the code easier to understand and adapt in the future. Moreover, it helps in ensuring that each part of the transformation can be independently modified without disrupting the entire process.
The key to successful data processing with Laravel pipelines lies in defining each “pipe” in a way that is self-contained and has a single responsibility. This approach not only makes the process more accessible and maintainable but also allows for better testing practices. Different “pipes” can be mocked or tested independently to ensure that they correctly perform the intended operation on the data.
Even sophisticated processes, such as dynamic report generation or complex filtering logic, can be streamlined using pipelines. Each requirement can be segmented into individual tasks and subsequently passed through the pipeline for an elegant, maintainable solution. By leveraging the power of Laravel pipelines, developers unlock the potential of a streamlined approach to intricate data handling.
Optimizing Performance with Laravel Pipelines
Performance optimization is another area where Laravel pipelines can shine. Since pipelines process tasks sequentially, it’s crucial to ensure that each “pipe” is as efficient as possible to avoid bottlenecks. Profiling the application to identify slow-running segments allows developers to fine-tune individual pipes for better performance.
One effective optimization technique is to limit the number of database queries within your pipes. Queries should be well-crafted and, where possible, eager loaded to prevent the N+1 problem that can significantly slow down data processing. Additionally, caching results within pipelines is an excellent strategy for avoiding unnecessary computations and database hits.
It’s also beneficial to leverage the lazy collection feature in Laravel when dealing with large datasets. Lazy collections allow for memory-efficient iteration through huge data sets by only fetching one item at a time. Incorporating this feature into pipelines can drastically reduce memory usage and improve execution speed.
Overall, Laravel pipelines provide an elegant and flexible system for handling a range of tasks within an application. When used correctly, they enable developers to create cleaner, more maintainable code that can be easily expanded and optimized. By adhering to best practices and being aware of common pitfalls, one can take full advantage of the simplicity and power that Laravel pipelines offer.